Using hooks with react-redux
August 26, 2020
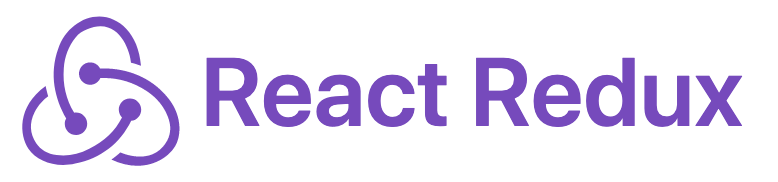
Official documentation for react-redux hooks can be found here.
In these examples, I'm using redux-thunk to call actions as functions instead of calling the type and payload.
The useSelector and useDispatch hooks are used in the same fashion as the hooks provided by React. The useSelector hook takes an arrow function that is passed a state object which is the entire global state of the store. You select which part of that state to return.
useDispatch is assigned to a variable and then can be called anywhere that you need to dispatch an action.
Using react-redux hooks is a lot cleaner because you don't have to connect the component, use mapStateToProps or pass actions through props. This makes development with TypeScript easier.